조건문과 반복문
if
weather = input('오늘 날씨는 어때요? ') # input을 이용해 다음과 같이 작성 후 실행 시 커서가 ? 뒤에서 기다리고 있다. 사용자가 입력한 값은 문자열로 저장된다.
if weather == '비' or weather == '눈':
print('우산을 챙기세요.')
elif weather == '미세먼지':
print('마스크를 챙기세요.')
else:
print('준비물이 필요 없어요.')
temp = int(input('기온은 어때요? ')) # 정수형으로 입력값을 받고 싶을 때
if 30 <= temp:
print('너무 더워요, 나가지 마세요.')
elif 10 <= temp and temp < 30:
print('괜찮은 날씨에요.')
elif 0 <= temp < 10:
print('외투를 챙기세요.')
else:
print('너무 추워요, 나가지 마세요.')
if 문을 통해 원하는 조건에 맞는 코드를 실행시킬 수 있다. 그리고 만약 처음 if의 조건에 성립하지 못했다면 elif를 만나고, 이러한 if 와 elif 모두의 조건에 성립할 수 없다면 else로 넘어가게 된다.
위 코드에서 쓰인 input을 통해서 사용자가 입력한 입력값을 받을 수 있다.
for
for waiting_no in [0, 1, 2, 3, 4]:
print("대기번호: {0}".format(waiting_no))
for waiting_no in range(5): # 0 부터 5 직전까지
print('대기번호: {0}'.format(waiting_no))
for waiting_no in range(1, 5): # 1부터 5 직전까지
print('대기번호: {0}'.format(waiting_no))
starbucks = ['아이언맨', '토르', '아이엠 그루트']
for customer in starbucks:
print('{0}, 커피가 준비되었습니다.'.format(customer))
for문을 통해서 원하는 만큼 코드를 반복적으로 실행할 수 있다.
students = [1, 2, 3, 4, 5]
print(students)
students = [i + 100 for i in students] # students의 각 요소를 i로 불러오면서 그 i에 100 더한 값을 students 리스트에 넣어라.
print(students)
students = ['Iron man', 'Thor', 'I am groot']
print(students)
students = [len(i) for i in students]
print(students)
students = ['Iron man', 'Thor', 'I am groot']
print(students)
students = [i.upper() for i in students]
print(students)
이렇게 리스트 안에서 반복을 진행하여 각 요소의 값을 바꿀 수 있다. 첫 번째 students는 [101, 102, 103, 104, 105]가 되었을 것이다. 두 번째 students 리스트는 각 요소가 길이로 반환되어 [8, 4 ,10]이 된다. 세 번째 students는 모두 대문자로 바뀌게 된다.
while
customer = '토르'
index = 5
while index >= 1:
print("{0}님, 커피가 준비 되었습니다. {1}번 남았습니다.".format(customer, index))
index -= 1
if index == 0:
print('커피는 폐기처분되었습니다.')
while 문은 조건을 만족하는 한, 계속해서 while 밑의 코드가 실행된다. 위 코드는 index가 점차 5, 4, 3, 2, 1로 줄어들면서 5번 반복하게 되고 0이 되면서 index >= 1을 만족하지 못하게 되기 때문에 while문이 종료된다.
customer = '아이언맨'
index = 1
while True:
print('{0}님, 커피가 준비되었습니다. 호출 {1}번 했습니다.'.format(customer, index))
index += 1
이처럼 코드를 작성 시, 계속해서 True이기 때문에 무한 루프에 빠지게 된다. 터미널에서 index가 계속해서 증가하는 것을 목격할 수 있다.
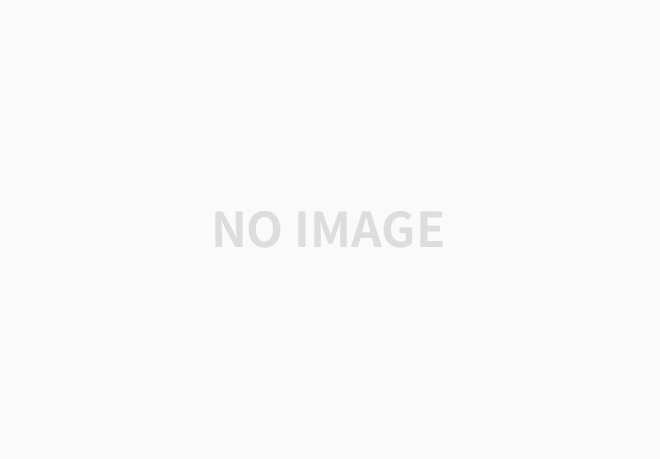
customer = '토르'
person = 'Unknown'
while person != customer:
print('{0}님, 커피가 준비되었습니다.'.format(customer))
person = input('이름이 어떻게 되시나요? ')
if person == '토르':
print('네, 감사합니다.')
위 코드에서는, input에서 사용자가 토르라고 입력하지 않으면 while문이 계속해서 실행된다. 사용자가 토르라고 입력하면 person = '토르'가 되고 if문을 실행하여 '네, 감사합니다.'를 실행시킨다. 그리고 person != customer는 False가 되어 while이 종료된다.
continue, break
absent = [2, 5]
noBook = [7]
for student in range(1, 11):
if student in absent:
continue # continue를 만나면 밑에 있는 문장을 실행하지 않고 다음으로 넘어간다. 즉 2나 5에서는 책 좀 읽어줘를 실행하지 않고 3과 6으로 넘어가게 된다.
elif student in noBook:
print('오늘 수업은 여기까지. {0}은 교무실로 따라와'.format(student))
break # break를 만나면 곧바로 반복문이 종료된다. 즉 7에서는 8로 넘어가는 것이 아니라 반복문을 종료시킨다.
print('{0}, 책 좀 읽어줘.'.format(student))
continue를 만나면 밑의 코드를 무시하고 다음 차례로 넘어간다. 즉 if문에서 현재 student가 absent와 동일한 2라면 continue가 실행된다. 따라서 '책 좀 읽어줘'라는 print문이 실행되지 않고 student는 3으로 넘어가게 된다.
break를 만나면 반복문을 종료시키게 된다. 만약 현재 student가 noBook과 동일한 7이라면 elif문의 코드가 실행되고 print문이 실행되면서 while이 멈추게 된다. break는 다음 차례로 넘어가는 continue와는 다르게 8로 넘어가지 않고 7에서 for문을 종료한다는 것이다.
퀴즈
# while 사용
currPassenger = 1
passCount = 0
while currPassenger < 51:
leadTime = randint(5, 50)
if 5 <= leadTime <= 15:
print('[O] {0}번째 손님 (소요시간 : {1})'.format(currPassenger, leadTime))
passCount += 1
else:
print('[ ] {0}번째 손님 (소요시간 : {1})'.format(currPassenger, leadTime))
currPassenger += 1
print('총 탑승 승객 : {0} 분'.format(passCount))
count = 0
for i in range(1, 51):
leadTime = randrange(5, 51)
if 5 <= leadTime <= 15:
print('[O] {0}번째 손님 (소요시간 : {1})'.format(i, leadTime))
count += 1
else:
print('[ ] {0}번째 손님 (소요시간 : {1})'.format(i, leadTime))
print('총 탑승 승객 : {0} 분'.format(count))